
Fig. 1
Arduino 74HC595 Serial Shift Register Interfacing
Here we look into connecting a 74HC595 8-bit serial shift register with 8-bit latch to an Arduino Microcontroller. Fig. 1 shows the internal configuration.
While Arduino has built in commands for serial output by writing our own we can port the basic program to other platforms such as the Raspberry Pi.
// use just k instead of !k // for common cathode displays. void byteOut(byte j) { byte k; for(int i=0; i < 8; i++) { k = j & 0x01; digitalWrite(DATA, k); digitalWrite(CLK, HIGH); digitalWrite(CLK, LOW); j = j >> 1; } // latch byte digitalWrite(LATCH, HIGH); digitalWrite(LATCH, LOW); }
Above is our serial shift subroutine byteOut(). It's nothing more than a for loop, a bitwise AND, and right-shift that sets or clears the DATA pin, pulses (HIGH then LOW) the shift clock, then after doing that 8 times pulses (HIGH then LOW) the latch pin. Here we shift out the least significant bit first.
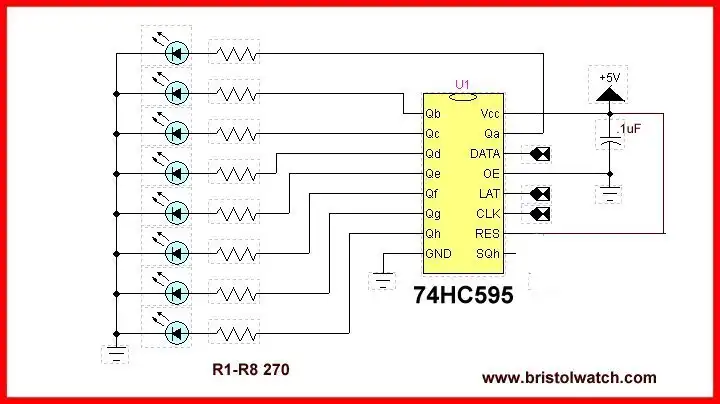
Fig. 2
Fig. 2 shows the LED connections. The LED pattern is simply a reproduction of the binary pattern shifted into the 8-bit register. In this case we simply count in binary from 0-255 right to left.
// Count 0-255 74HC595 #define DATA 8 // 74HC595 pin 14 #define LATCH 9 // 74HC595 pin 12 #define CLK 10 // 74HC595 pin 11 void setup() { pinMode(DATA, OUTPUT); pinMode(LATCH, OUTPUT); pinMode(CLK, OUTPUT); digitalWrite(DATA, 0); digitalWrite(LATCH, 0); digitalWrite(CLK, 0); } // end setup void loop() { for(int i=0; i<=255; i++) { byteOut(i); delay(200); } } //end loop // use just k instead of !k // for common cathode displays. void byteOut(byte j) { byte k; for(int i=0; i < 8; i++) { k = j & 0x01; digitalWrite(DATA, k); pulseCLK(); j = j >> 1; } pulseCS(); // latch byte } void pulseCLK() { digitalWrite(CLK, HIGH); digitalWrite(CLK, LOW); } void pulseCS() { digitalWrite(LATCH, HIGH); digitalWrite(LATCH, LOW); }
- Quick navigation of this website:
- Basic Electronics Learning and Projects
- Basic Solid State Component Projects
- Arduino Microcontroller Projects
- Raspberry Pi Electronics, Programming
- Electronics hacks:
- Connecting PCF8574P GPIO Expander to Raspberry Pi
- Programming PCF8574P 8-bit I-O Expander with Arduino
- Raspberry Pi MM5451 LED Display Driver
- Raspberry RTC with MAX7219 Display Driver
- Raspberry Pi 8-Digit LED MAX7219 Display Driver
- Raspberry Pi 74HC595 Serial Shift Register
- Interface I2C LCD to Raspberry Pi in C
- ADS1115 4-Channel ADC Uses I2C with Raspberry Pi
- MCP4725 12-Bit DAC Interface to Raspberry Pi
- WiringPi and Pulse-Width-Modulation with Raspberry Pi
- WiringPi for Raspberry Pi and MAX6675 thermal-couple sensor
- WiringPi Blink an LED Demo
- Simple GPIO Reference Box
- Raspberry Pi with PCF8591 Analog To Digital Control in C
- Raspberry Pi PCF8591 AD-DA Sensor Python Interface
Web site Copyright Lewis Loflin, All rights reserved.
If using this material on another site, please provide a link back to my site.